An address autocomplete is an address form feature that’s kind of just what it says on the tin: it automatically suggests addresses to users as they type into an online form. It’s an elegant way to ensure accuracy as a user inputs all the components of a complete address, like country, city, postal code, and street.
But building a form to capture an address with auto-complete and validation can be quite challenging. What should the form ask for first: the postal code or the region? Which data input should use auto-complete? Which reliable data source should be used? How to optimize UI responsiveness?
In this article, you will learn all the steps required to build a backend API for address completion.
The technicalities of building an API backend and frontend are beyond the scope of this article. They’re too dependent on your chosen programming language. As a personal recommendation, I favor React with Material UI for the frontend and Node.js with Express for the backend. But you will need some solid technical knowledge about building an API backend and frontend before you read on.
What Does an Address Autocomplete Feature Actually Look Like?
To expound on my earlier definition, an address autocomplete feature generates suggestions based on previously entered information as the user enters data into the form. The user can easily choose from a list of options, ensuring the accuracy of the input address. This is especially useful when someone needs to enter a long or complex address or when the user isn’t familiar with a specific address format.
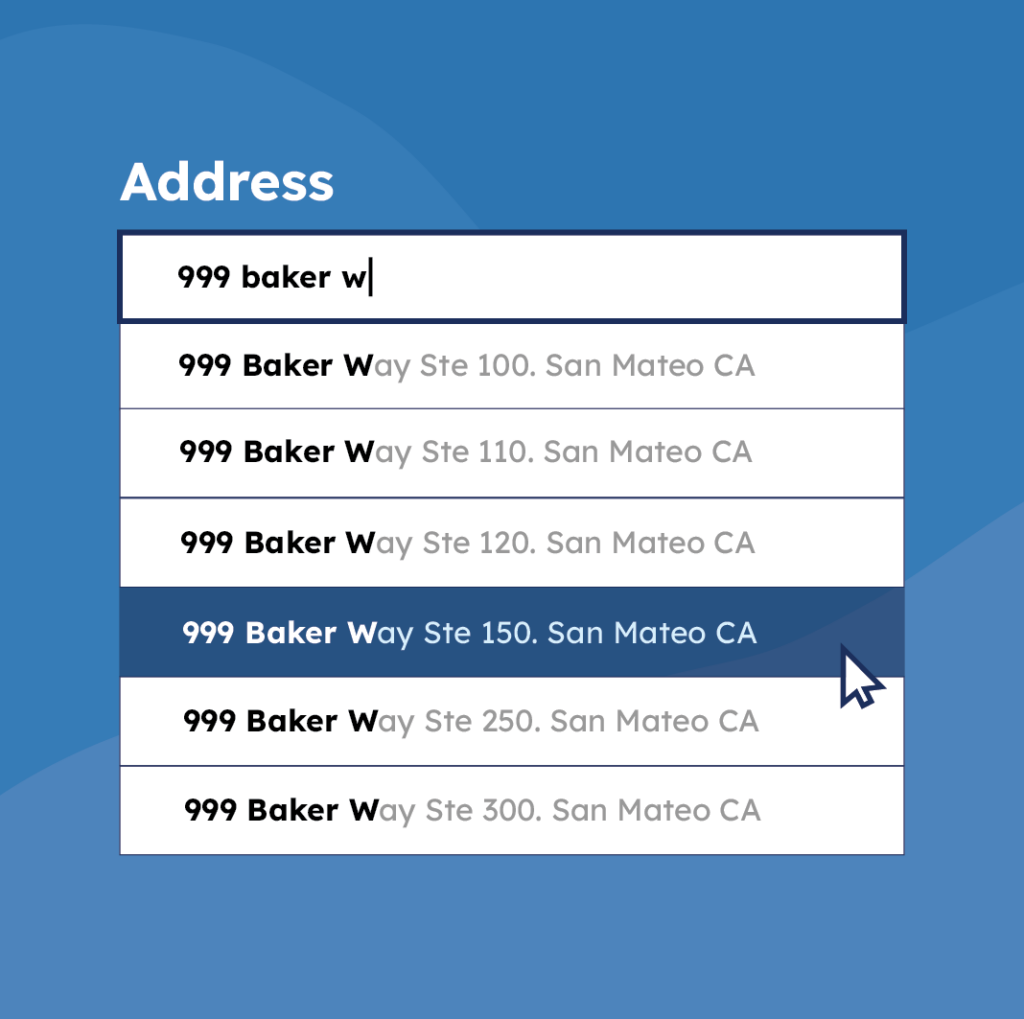
Why Is Address Autocomplete Important?
An efficient address autocompletion form on your website prevents you from ingesting inaccurate data, like typos or nonexistent addresses. Unless you allow free entry, you could also lose sign-ups or orders. But allowing that will even worsen the data quality.
Critical Role in Data Quality
Ensuring data quality right from the start is critical for proper data processing. Without it, you risk having incorrect shipping addresses, which can cause delays, extra costs, warnings in your billing system, or incorrect recommendations from a store locator feature—all bad for your business and user experience.
Positive Impact and Benefits
We’ll explore the benefits of Address Autocompletion, from enhancing user experience to improving data quality and operational efficiency.
Easier User Experience
But the feature isn’t just about preventing negative events. Address autocompletion can have quite a positive impact simply by making things easier on your users, like when someone has a time constraint and needs to complete a purchase quickly.
Enhanced Data Quality and Operational Efficiency
It will also increase the overall quality of all the addresses you collect in your database, decreasing your operational costs.
Building and Implementing an Address Autocomplete API
For this article, I’m going to assume that you’re using a data source with a structure similar to this:
Countries
Lists all countries and their corresponding ISO code. This table should also include metadata cataloging which data is present or not, like streets that might be missing or whether no postal codes exist for a certain country.
Places
Linked to a country, lists the cities and towns.
Postal codes
Linked to a place, lists the related postal codes. Note that one postal code can be included in more than one place.
Streets
Linked to a place and a postal code, lists all related streets. Note that the same street can cross multiple places or postal codes.
Keep a Few Tips in Mind
Keep a few tips in mind as we explore managing house and apartment numbers, handling missing data, and leveraging a backend API for address validation and autocomplete functionality.
House and Apartment Numbers
For building and apartment numbers, I work on the assumption that the data source doesn’t provide accurate information. Having a complete and accurate source of house numbers is difficult, so I advise you to leave that as a free input. Otherwise, your captured data quality will probably be impaired.
Handling Missing Data
Note that some countries don’t have postal codes, or your data source might not have accurate data for that country. In that case, show a free input for the fields that are missing data.
Backend API and Referential Database
The form built in this tutorial calls a backend API that’s linked to a referential database. The API sends back and validates the data from the source and provides autocomplete when necessary. It’s important to remember that the quality of the result depends greatly on the quality of the reference data source. You can read the paragraph Ensuring the Quality of Your Datasource to learn more about this.
Choose Your Field Order
There are two possible ways to approach building this form. You can enforce the fields to fill in this order:
1. Country -> Postal code -> Locality -> Street. This approach is easier technically, but some users might prefer to see a filtered list of postal codes.
2. Country -> Locality -> Postal code -> Street. This is a more traditional approach.
I recommend combining the two approaches in one form and letting the user fill in whichever field they want first. The country should still be enforced as the first field to fill because it’s information all the users can give quickly and correctly. It also narrows the possible choices and allows you to hide or show some fields. As mentioned earlier, some countries don’t even have postal codes, so you wouldn’t need to show a field for them.
The final result for Approach 1 should look like this:

The final result for Approach 2 should look like this:

Display the Data in the Form
For some endpoints, the data is returned based on what the user is currently typing. To avoid having too many hits on your API endpoint, I recommend a delay of 20 ms. In that time, the user might continue typing their query; by introducing a delay, you avoid sending useless queries to the backend.
A convenient way to implement that functionality is to use the RxJS debounceTime feature.
Build the API
Here I’ll describe all the endpoints you need to serve to have a complete API. But first, let me share a few quick insights that I’ve found helpful:
Parameter Reuse in Queries
If a path contains a colon (:
), that indicates a parameter that will be reused in the query.
Content Structure for POST Requests
For POST requests, the content’s structure is described as the payload in a JSON format.
Autocomplete Endpoint Considerations
- For all the API endpoints doing an autocomplete, don’t return anything if the user hasn’t started typing. It would make little sense in terms of user experience.
- When filtering on a user’s input, always do a case-insensitive search with wildcards before and after
- The queries that might return a lot of data should be limited to 100 results to increase the query performance and the response time.
Unique Identifiers and Licensing
- Consider that important entities like postal codes and places will have a unique identifier. Depending on your data source, the structure might differ slightly.
- Depending on your data source’s license, you might want to implement a per-user throttling and quota mechanism to avoid scrapping all the data.
Approaches 1 and 2 — Country
Endpoint: GET /countries
This returns the list of countries without any filtering. The list is short to be loaded entirely on the client side.
Again, remember that some countries don’t have postal codes, and your data source might not have street data for all the countries. This is a good place to return that information and show a free text field for the missing data.
Approach 1 — Postal codes from Country
Endpoint: GET /countries/:iso/postcodes/:postcode
This returns the autocompletion of a postal code for a given country. The data must be filtered on the postal code.
Approach 1 — Places from Postcode
Endpoint: GET /postcodes/:postcodeId/places
Given a postal code, the API will return all the associated places.
Approach 2 — Places from Country
Endpoint: POST /countries/:iso/places
Payload: { place }
This query should return all the places in a country. The data is filtered on the place entered by the user.
Approach 2 — Postal codes from Place
Endpoint: GET /places/:placeId/postcodes/:postcode
If the user chose a place from a country, this endpoint must be used to autocomplete the postal code.
Approach 1 and 2 — Street from Place
Endpoint: POST /places/:placeId/streets
Payload: { postcodeId, street }
Note that the place and the postal code should be necessary to filter the street correctly. This of course, depends on your data source, but combining both usually offers the most accurate discriminator.
Improving Your Address Autocomplete API
The autocomplete mechanism presented in this article only works on perfect matches. That’s rarely good enough, so you’ll likely want to improve on what we’ve already got here.
Implement Full Text Search
Users are going to make spelling mistakes. To alleviate that in simple projects, you can use trigrams or the full-text search capability of your DBMS.
For more complex solutions, you can use a dedicated search engine such as Elasticsearch, OpenSearch, or Typesense.
Use Additional Datasets
Additional data can significantly enhance the user experience.
Weighted Data for Auto-completion
For example, consider how weighted data can improve the auto-completion. The most important places have a heavier weight, which would sort the results in a comprehensible order for the user while aiding in disambiguation. With correctly weighted data, Boston (Massachusetts) would appear first in a list of the many cities named Boston in the United States.
Grouping Places for User Expectations
Another interesting improvement would be grouping places by the name people expect to find. For example, London doesn’t actually exist as a place within the fine-grained English administrative divisions but is rather a group of boroughs. Your users will probably still be looking for London
, though.
Ensuring the Quality of Your Datasource
The quality of the data source used to build your API is critical to the success of your autocomplete feature. Otherwise, you’ll gather incomplete information at best and inaccurate information at worst. If a user enters data not present in your dataset, and you’ve made the use of your data mandatory, you could even lose the sign-up or the order, decreasing your overall conversion rates. This can be mitigated by allowing free text to be entered, but will worsen the captured data quality.
Data Source Requirements
The data source you work with needs to include an up-to-date, exhaustive list of valid postal codes and places for all your target countries. Streets can be left optional, but having a complete list of streets helps. As it’s difficult always to have an up-to-date list of valid addresses, it’s best to allow free text. You can combine both approaches by trying auto-complete first and then allowing free text if nothing is found.
Options for Data Sources
You’ve got 2 main options for your sources.
Free or Open-Source Data Providers
If you’d like to use free or open-source data providers, we recommend combining GeoNames and OpenStreetMap. GeoNames links places to postal codes for 96 countries, while OpenStreetMap offers street coverage for most countries.
Paid Solution
If you want to invest in a paid solution, I recommend our GeoPostcodes database. It contains places for all countries in the world, postal codes for all countries using them, and streets for a third of the countries. It’s updated every week, relying on more than 1,500 sources. You would benefit from the fact that postal codes and streets are already linked in a unified structure. Based on our experience, it would increase the quality of your API and its speed of implementation. Browse our datasets yourself and access our map explorer for free.
The API described in this tutorial would benefit from GeoPostcode products like:
- Our postal normalized street database: It includes alternative place names in foreign languages.
- City ranking: This improves results sorting by putting the most relevant city first.
- City tags: You can natively incorporate large cities and metropoles in the search.
Importance of Autocomplete Address Form
Having an autocomplete address form is crucial to many business processes, like logistics or online sales. It vastly improves data quality and ensures correct data processing. With all the information covered here—what it looks like to start with the postal code or place for your form and why you’ve got to have a reliable and updated data source—you should be well on your way to creating the perfect form to capture accurate addresses.
FAQ
What is autocomplete address?
An address autocomplete is a functionality used in online forms and mapping applications to assist users in entering addresses in the right format.
When a user types an address in a form field or address search bar, the address autocomplete system suggests potential matching results based on relevance or proximity to the user’s location.
What is a global address autocomplete API and how does it ensure correct address input?
A global address autocomplete API is a tool designed to streamline the address input process by suggesting valid addresses in real-time as users type. By leveraging local postal authority formats, this API enhances address data quality and verifies the accuracy of addresses entered into address forms. Businesses can customize the API to match specific address forms, ensuring that users input correct addresses according to the local postal authority format for a valid address.
How can businesses enhance address data quality with address autocomplete and verification tools?
Businesses can enhance address data quality by implementing address autocomplete and verification tools. These tools suggest valid addresses in real-time and verify them against local postal authority formats, ensuring the accuracy of addresses collected through online forms. By prioritizing address data quality, businesses reduce errors and improve the reliability of their address databases, thus ensuring they collect valid addresses.
What role does local postal authority format play in address verification?
Local postal authority format plays a crucial role in address verification as it serves as the standard for valid addresses in a specific region. Address verification tools compare input addresses against local postal authority formats to ensure correctness and validity. By adhering to the local postal authority format, businesses can optimize address data quality and reduce errors in address submission, ensuring they collect valid addresses.
Can address autocomplete APIs be customized for different address forms?
Yes, address autocomplete APIs can be customized to match the requirements of different address forms used by businesses. By tailoring the API to specific address formats, businesses can ensure that users input correct addresses in the local postal authority format. Customization options enable businesses to optimize address autocomplete for various applications while maintaining address data quality and ensuring valid addresses are collected.
How does address autocomplete API streamline the address input process?
ddress autocomplete API streamlines the address input process by suggesting valid addresses as users type. This real-time suggestion feature reduces the likelihood of errors and incomplete submissions, thereby enhancing address data quality. By offering accurate suggestions based on the local postal authority format for a valid address, address autocomplete API improves user experience and efficiency in address input.
How does an autocomplete address API assist user in inputting street address?
An autocomplete address API helps users input street addresses accurately by suggesting valid options as they type. By leveraging advanced algorithms and integrating with mapping services, this tool streamlines the process of entering street addresses, ensuring that users can quickly find and select the correct option.
Can a user input address manually when using an autocomplete address API?
Yes, users have the option to input addresses manually when using an autocomplete address API. This allows users to enter addresses directly, bypassing the autocomplete suggestions if desired. Whether pinpointing a location on a map or specifying a specific address, manual input offers flexibility and ensures accurate address information.
How does Google Map enhance the functionality of an autocomplete address API?
Google Maps enhances the functionality of an autocomplete address API by providing maps and location data. By integrating with Google Maps, an autocomplete address API ensures that users can easily find and select addresses from the map, further improving the accuracy and relevance of address suggestions.